How to Use Moment.js in React
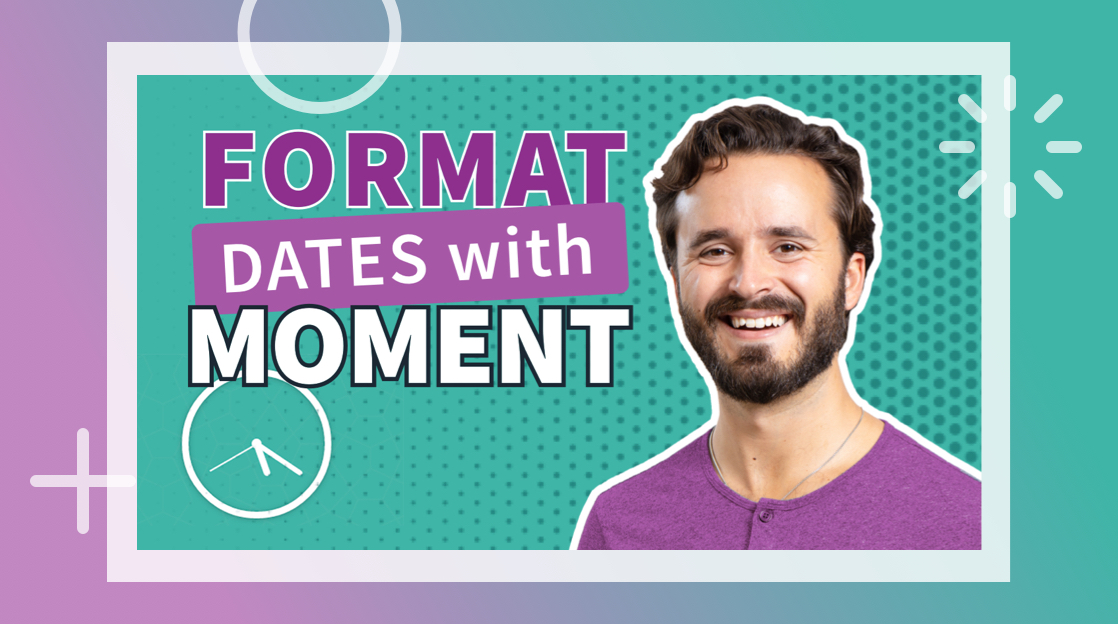
Have you ever tried to make a date picker for React? It’s not an easy task. Understanding how to figure out how many days are in a month in JavaScript can be a nightmare. Sure, the Date function helps a little, but using Date in JS to get how many days are in a month requires a lot of extra leg work.
There’s an easier way, however. A library called Moment.js makes things like figuring out if it’s the second Tuesday of the month easy as pie. Let’s work through this Moment.js tutorial and explain how Moment.js in React can make your apps easier to write.
An Overview of How to Use Moment.js in React [VIDEO]
In this video, CBT Nuggets trainer Shaun Wassell walks through all the key functions within the Moment.js library that you will need to build a calendar app.
What is Moment.js?
Moment.js is a JavaScript library that helps parse, validate, and manipulate date objects in JavaScript. Moment.js can also be used with Node and other JavaScript frameworks like React, Vue, and Angular.
How to Use Moment.js
Moment.js is easy to use. First, let’s explain some essential functions included with Moment.js. Then, we’ll combine these functions to determine how many Tuesdays are in the month.
Before working Moment.js, it needs to be imported and declared in React:
import moment from"moment";
Once Moment is imported, set your preferred data format. In this case, we’ll use American standards:
moment().format("MM/DD/YYYY");
How to Parse a Date in JavaScript
The date of the Moment object can be used to parse a date, too:
moment("01/01/1999", "MM/DD/YYYY");
Of course, setting the date and date format for a Moment object isn’t very helpful without storing it as a variable to work with later:
constaLongTimeAgo=moment("01/01/1999", "MM/DD/YYYY");
How to See How Many Days Are in a Month in React
Once a new instance of Moment is instantiated, we can use other Moment functions to figure out what the current month is, how many days are in that month, and more.
// Get the current month
aLongTimeAgo.month();
// Get the total days in the month
aLongTimeAgo.daysInMonth();
We can also add and subtract days from the current Moment object.
// Get five days in the future
aLongTimeAgo.add("5", "days");
// Get five days in the past
aLongTimeAgo.subtract("5", "days");
How to See the First Day of the Month in JavaScript
Here is how to get the first day of the month in Moment.
// Get start of month
aLongTimeAgo.startOf("month");
It’s important to understand that when you manipulate the moment object, it mutates the object itself. Otherwise, if you add or subtract five days from the current Moment object, the new value for the Moment object changes and becomes five days in the future or past.
If the Moment object shouldn’t be mutated, it needs to be copied first.
// Clone moment
constnewMoment=aLongTimeAgo.clone();
How to Determine How Many Days Are in a Month in React
Now let’s combine all of this to figure out how many Tuesdays are in the current month in React.
import moment from"moment";
constgetDaysInMonth= (monthMoment) => {
constmonthCopy=monthMoment.clone();
monthCopy.startOf("month");
let days = [];
while (monthCopy.month() ===monthMoment.month()) {
days.push(monthCopy.clone());
monthCopy.add(1, "days");
}
return days;
};
constdays=getDaysInMonth(moment());
console.log("days:", days);
First, define an arrow function passing a Moment object to it. Once the arrow function is declared, clone the Moment object. We don’t want to mutate the current object. After cloning the object, set the cloned copy to the first day of the month. Next, define an empty array. Then loop through each day of the cloned copy of the Moment object and store each day’s value in the array we just defined. Finally, return the array and print it to the console.
Look through the array printed in the console. You’ll notice that one of the values for each object in the array includes a timestamp with the conical name for the day of the week. Figuring out how many Tuesdays are in the current month is as simple as looping through the array and counting each Tuesday.
Go ahead and write a function that counts the number of Tuesdays in the month. This is an excellent exercise to pull everything you learned in this tutorial together.
Start Learning How to Use Moment.js in React
We’ve learned how to use Moment.js in React to work with dates more efficiently than using the built-in Date functions in JavaScript. How do you use Moment.js to create a custom datepicker in React and other JavaScript frameworks, though?
Check out Shaun Wassell’s React Datepicker from scratch tutorial at CBT Nuggets. Datepickers are a critical yet mundane feature of most applications, yet they can introduce tons of bugs if they aren’t implemented correctly. The logic that controls Datepickers can be incredibly tedious. Shaun does a deep dive into Moment.js and explains how to write a perfect, customized Datepicker for your JavaScript application.
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.