How to Compare Two Strings in JavaScript
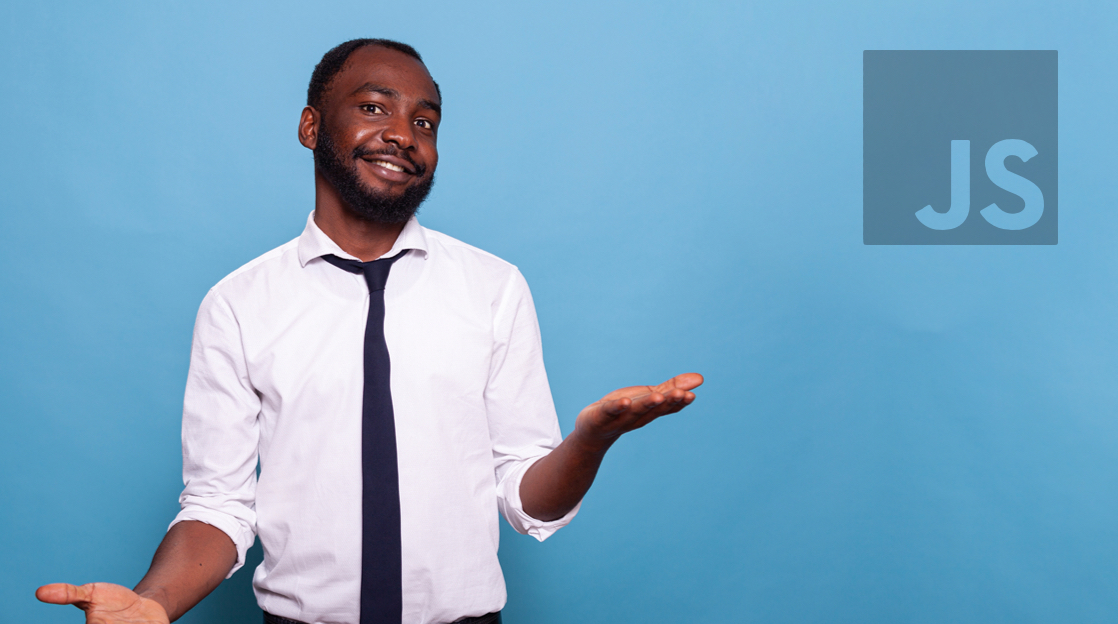
How do you compare strings in JavaScript? For humans, this process is easy. We automatically understand the nuances in language and slang. Like all programming languages, JavaScript is very literal, though. Javascript doesn't have comprehension skills like people do.
There’s a big difference between “Let’s eat grandma” and “Let’s eat, grandma” in the JS world. Comparing strings in JavaScript isn’t as straightforward as you might think. So, how does the robot know the difference? Let’s find out.
If you're looking for a more advanced intermediate JavaScript tutorial, go check out Shaun Wassell’s training. He teaches the ins and outs of working with strings in JavaScript by building a simple game.
How Do Strings Work In JavaScript?
In JavaScript, strings are sequences of characters enclosed in single (' ') or double (" ") quotation marks. Strings are one of the fundamental data types in JavaScript and are used to represent text or sequences of characters.
Strings are also immutable. Once a string is created, it cannot be changed. Using string-specific functions, such as .toUpperCase() or .replaceAll(), creates a copy of the original string. Unless that copy is assigned to a variable, it’s lost.
JavaScript strings are very similar to arrays. In fact, array functions can be used on strings in JavaScript. Here’s an example.
First, let’s define a string:
const example_string = “Lets eat grandma”;
We can use the native .length array function to see how big that array is. Likewise, bracket notation can be used on the string to see which character is in the third position of that string.
console.log(“example_string length: “, example_string.length);
console.log(“Third character in string: “,example_string[3]);
The syntax above should output the following to the console:
example_string length: 16
Third character in the string: t
It should be noted that JavaScript strings are basically arrays under the hood. However, JavaScript handles strings slightly differently than arrays because strings have an expected data type. Arrays, on the other hand, can have multiple data types. Because JavaScript has this built-in expectation, JavaScript treats strings slightly differently than arrays in some cases.
Explaining the Difficulties of Working With Strings in JavaScript
Working with arrays in JavaScript is typically easy. Arrays have specific values and predetermined characteristics. However, strings are more complicated. Strings typically receive values from user input. Humans are ambiguous, and language is messy. These nuances make strings messy as well.
As humans, we comprehend the difference between language and slang. We inherently know whether two sentences or statements are equal despite verbiage or punctuation.
JavaScript doesn’t comprehend equalities or similarities in something as complex as human language. JavaScript needs a little hand-holding.
There is a big difference between “Let's eat Grandma”, “Let's Eat Grandma”, and “Let's eat, grandma” in JavaScript. All three of those strings are different in the eyes of JavaScript, and using the equal operator against all three will return a false value. Though, to humans, all three sentences are the same.
Something as simple as capitalization or punctuation can make strings challenging to compare.
Ways to Compare Strings in Javascript
There are many ways to compare strings in JavaScript. Before explaining how to compare strings, let’s demonstrate why a simple equality operator doesn’t work.
The JavaScript statement in the example above returns false. The equality operator will compare all the values in the string to see if they match. Since strings in JavaScript are arrays, and some letters are capitalized while others are not, both strings are not equal.
That should be an easy fix, right? We could use the .toUpper() or .toLower() string functions to convert all the characters in both strings to equal values. There is an easier way, though. Use .localCompare() instead.
The .localCompare() function accounts for differences in capitalization. The example above will output “The strings are similar” in the console.
However, .localCompare() doesn’t account for additional spaces or punctuation in the string.
Notice the extra space between ‘eat’ and ‘grandma’ in the example above. The code in that example will print “The strings are not similar” to the console.
There is an easy way to fix this, too. We remove all punctuation and spaces from both strings first.
The example above uses the .replaceAll() string function to remove spaces from both strings and the comma from string4. The drawback is that a .replaceAll() call needs to be used for each type of character in both strings. If one of the strings includes a question mark, an additional line must be added to the code in the example screenshot above to account for that.
By removing all spaces and commas in both strings, the .localeCompare() function identifies both as equal.
Ready to Learn More About JavaScript Strings?
Comparing strings in JavaScript is that easy. This process could be better, however. For example, the .replaceAll() function can accept regular expressions, and by using a regular expression, all spaces, punctuation marks, and other random characters can be removed from strings with one command. We can also replace individual characters in strings and modify the final value as needed.
If you’re ready to learn more, sign up for Shaun Wassell’s Intermediate JavaScript Tutorial training. Shaun walks students through building a word game in JavaScript. You’ll learn how to handle the five million edge cases of working with strings in JavaScript by creating your own game. So, go get started!
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.