Why use Functions in Python?

Python is one of the most popular programming languages. It’s relatively easy to use, yet extremely versatile and powerful. Heck, it’s even the preferred language for data scientists. If you’re curious about Python, learning how to write Python functions is a good starting point. Today, we’ll explain what a Python function is and how to use them.
If you’re looking for a complete Python function class, go subscribe to John McGovern’s Python function tutorial instead of reading this article. He explains everything you need to know about creating well-scoped, proper functions in Python
An Overview of Writing Python Functions
In this video, CBT Nuggets training John McGovern introduces you to functions in Python — including how they work and how to write them.
How to Write a Python Function
Writing Python functions is fairly easy. A function is nothing more than a block of code. One of the best reasons to write Python functions is to separate reusable code from the core app so you don’t repeat yourself. A classic example of a chunk of reusable code turned into a function is one that calculates the square of a number. It’s boring, but it’s easy to understand.
A Python function consists of three core components:
A def statement defining a function
The code bits in the middle of the function that does something (in this case, finds the square of a number)
A return statement that ends the function
Python always expects a return value. That said, you don’t always need to add a return statement to your Python function. If the function doesn’t define a return statement, the function returns a default value of ‘none.’ Note that ‘none’ does not mean ‘null.’ Don’t confuse the two.
Here’s what our square function looks like in Python:
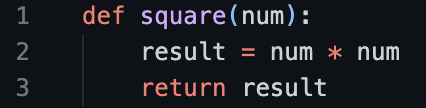
Python functions are that simple to write. First, the Python function is defined using the ‘def’ keyword. Immediately after the ‘def’ syntax is the name of the function. In this case, we called it ‘square.’ After giving our creation a name, we define what arguments the Python function accepts, followed by a colon that states where the Python function starts.
A Python function can accept more than one argument, but because we are simply calculating the square of a number, we only need one argument in this case. That’s because the square of a number is calculated by multiplying that number by itself.
With that said, the first line of this Python function defines a new variable with the square value of the number passed into the function (calculated by multiplying that number by itself). Don’t forget to indent the lines of code inside the Python function.
Finally, the square of the number passed into the function is returned to whatever called the Python function to begin with.
Congrats! You just re-created a basic math function already included in Python.
Why Should You Use Python Functions in Apps
Python functions are nothing more than blocks of code designed for specific purposes, but nonetheless, functions are an essential paradigm in programming. Despite each language needing to be unique, whether it’s called a function or a method, they all serve the same purposes.
1. Python Functions and the DRY Principle
One of those purposes is to adhere to the DRY principle. DRY means ‘don’t repeat yourself.’ As you’ll discover in the section below, bits of code should never be repeated. If a block of code is used multiple times in your app or script, turn it into a function. Stop repeating yourself.
2. Python Functions Provide Clarity
Another reason to write functions is clarity. Reading through hundreds or thousands of lines of code at a shot is very obnoxious. It’s like reading a book that doesn’t have any paragraphs. Would you like to read the source code for an app that looks like that? No!? Neither does anyone else. So, use functions to create clarity.
There are many reasons to write Python functions, but those are the two big ones. Hold both near and dear to your heart. Because if you upset senior developers, you will have a bad time. Don’t upset your leads, or you’ll be resigned to writing the most basic, tedious code for the rest of your career.
Start Learning Python Today!
Do you want a more detailed explanation of what Python functions are? Check out John McGovern’s CBT Nuggets Python Function Tutorial online training.
He’ll teach you how to write Python functions with best practices, explain the caveats of using functions in Python compared to other programming languages, and explain why Python functions make sense in procedural code used in things like network engineering.
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.