How to Design an Ideal React Form Component
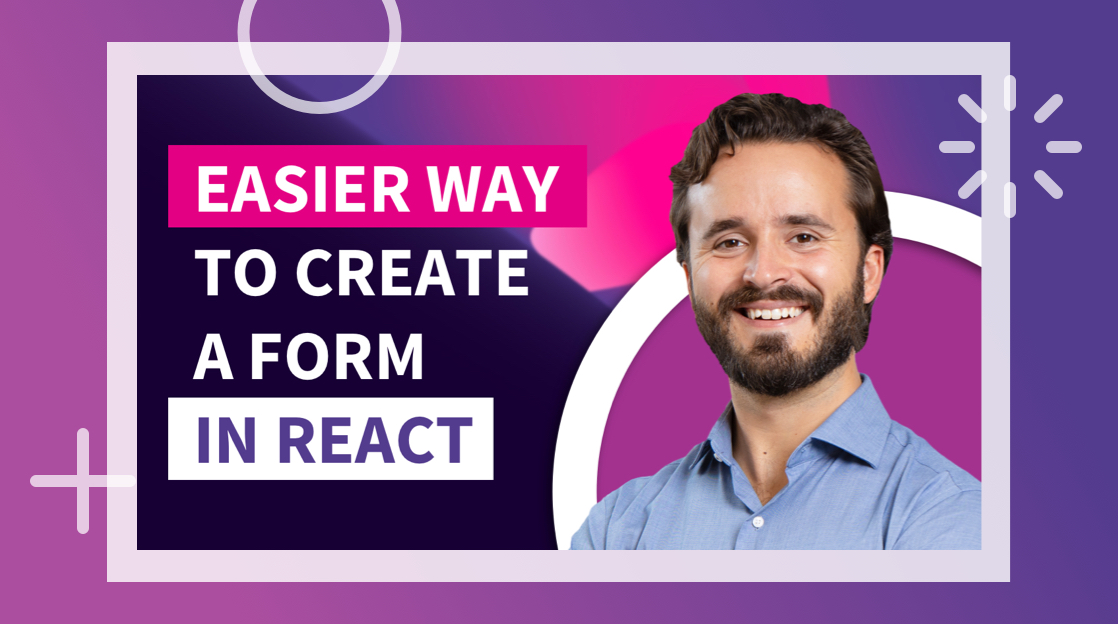
For most people, creating a React form involves invoking the state, defining inputs, and binding input values to the app state. Let me expand on that a bit for people that aren’t as familiar with React.
Single-page app (SPA) frameworks like React and Vue have something called a state. A state is a repository of values available throughout the entire app.
SPAs are built using different components. A component can be an entire page, like a login page, or something much smaller, like a button. Each component can also have a local state, except local states are only available to the specific component defining it – not the entire app.
Each component that uses the global state also needs to define which state values it wants to use. If we have variables in our global state defining user information (like usernames or email addresses), each component that wants access to that user information needs to call it. Calling the same state information in different components creates a lot of duplicate code. Likewise, if we create a React form to edit that user information on multiple pages, that form must be duplicated numerous times, too.
If you need a React form example demonstrating this, check out Shaun Wassell’s example in his React form tutorial.
An Overview of How to Design an Ideal React Form Component
In this video, CBT Nuggets Shaun Wassel shows how forms are typically developed in React – in all their inefficient, repetitive, tedious glory. Watch along with him as he shows you a more efficient, tidier, and reusable way to build forms inside React.
What are Reusable Components in React?
Single-page app frameworks like React depend on re-usability. If you’re familiar with object-oriented programming, use that as a metaphor. Components in React can be re-used.
Let’s look at this at a high level. Let’s say that you built the most fantastic audio editing app. A core component of an audio editing app is going to be an audio player. An audio editing app will most likely need access to that audio player on multiple pages.
However, you shouldn’t need to create that audio player more than once.
This is an excellent example of a reusable component in React. A single component contains one audio player that can be re-used on multiple pages in the SPA. Data is passed back and forth between components with something called a prop.
In object-oriented programming, this is like instantiating a new object and passing the values for that object to it when it is created. If the dashboard for your audio editing app needs to use an audio player, it might ‘instantiate’ that audio player component and tell it which audio file it needs to play via props.
How Can Components be Used to Make React Forms?
We can de-duplicate the code required to build a React form by building that form within a reusable component. This requires a bit of planning, however.
Reusable components need to be versatile. In this case, we have no idea what the React form in our component will be used for. That creates complications.
A React form can be used to log in, upload audio files, or purchase something online. Each one of those forms requires different types of inputs. We don’t want our React form to display a password input if we’re trying to upload an audio file, for example.
In this case, we want our React form to build itself appropriately. There are a few ways to do this, but all of them involve a bit of logic.
For instance, if we call our reusable React form component to create a login form, we could pass an array of values containing which inputs we need. For a login form, we’ll need inputs to enter a username and password at a minimum. So, our array passed to the React form component via props might include values telling the component to create username and password fields.
It’s the logic’s responsibility in our reusable component to parse the props passed to it and figure out which inputs we need. Likewise, all our React form validation logic is stored in the same reusable component, so we don’t need to duplicate it multiple times. That’s a bonus. Validation logic can get messy quickly.
Start Learning How to Use React Forms Today!
Using reusable components is good practice in React and every other SPA framework. Learn good habits now. Don’t learn how to do things the wrong way. It’s difficult to unlearn bad habits. Let Shaun Wassell be your guiding light. Shaun offers multiple React classes, including one specifically for designing reusable React forms. So go ahead and get started today!
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.