How to Add Firebase Auth to an Angular Application
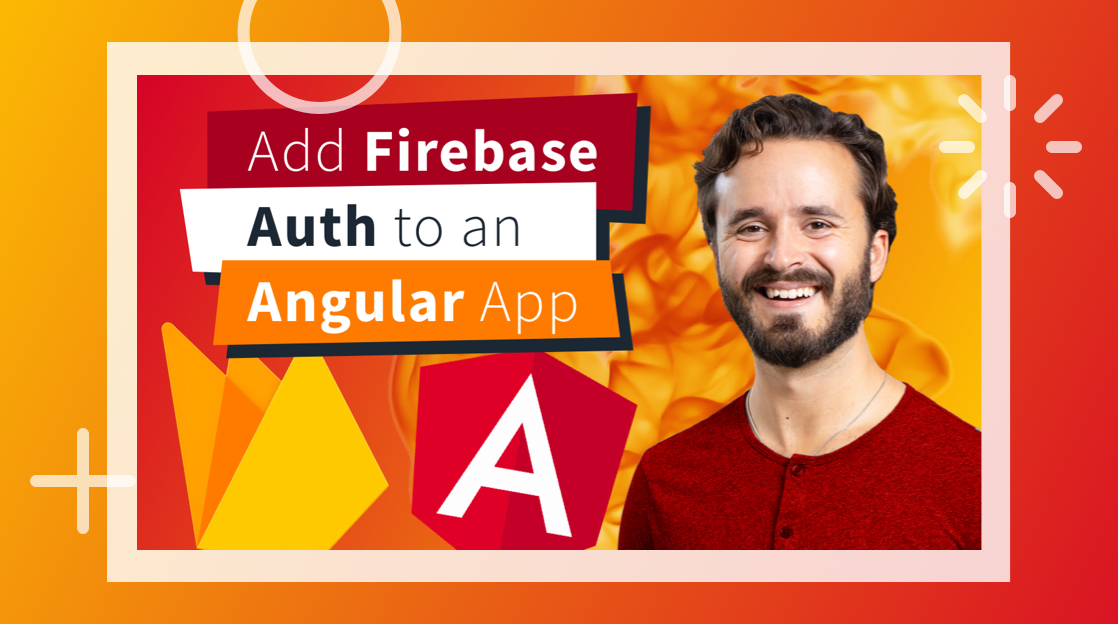
Wouldn’t it be nice to skip the whole process of creating user authorization services in your new Angular app? It’s a pain and it’s boring. But there’s a better way. Google provides a free service called Firebase. Firebase makes adding user authorization into an Angular app easy peasy. Best of all, the free tier for Firebase is fantastic.
We’re going to briefly describe the general process of adding Firebase Auth to your Angular app in this article. We assume you’re familiar with Angular and have built a couple of small apps with the guidance of other guides.
If you came here for a step-by-step tutorial, I recommend subscribing to Shaun Wassell’s Angular and Firebase Training online training. He goes much deeper into how Firebase integration works with Angular apps using best security practices.
An Overview of Adding Firebase Auth to Angular Applications
In this video, CBT Nuggets trainer Shaun Wassell covers integrating Firebase Auth into your Angular code. This video is for beginner coders. All you need to know is how to create a new Angular project and how to add pages to it — as well as what authentication is and why you need it.
How to Create the Firebase App and Add Keys to an Angular App
When you’re ready, head over to Firebase and log into the administrative account. Locate the option to create a new app. Follow the wizard presented after selecting that option. After a moment, a Firebase app will be configured and ready to go.
When the new app process is completed, Firebase will give you instructions for implementing Firebase into your app. Go ahead and read through each step if you haven’t in the past. While we won’t be using many of these steps, it’s good to understand the typical integration process.
For now, copy the six lines in the Firebase config settings – specifically, copy the apiKey line to the appID line. Once shoved into your clipboard, open the environments.ts file in your Angular app. Create a new key in the Environments file called Firebase, and paste the copied lines as their values.
It should look something like this:
export const environment = {
production: false,
firebase: {
apiKey: …
}
}
How to Install Firebase Packages
Now it’s time to add the proper Firebase libraries to your Angular app. In this case, we only need two. One of the libraries is a Firebase implementation specifically for Angular. The other is the Firebase library itself.
When you’re ready, open a terminal window and navigate to the root folder of your Angular app. Next, use NPM to install both packages mentioned above. The specific command you need is ‘npm install @angular/fire firebase.’
Note that we didn’t target a specific version of Firebase in that example. It’s always a good idea to specify which package version you want for production apps. That way, packages don’t update accidentally and introduce new problems.
Once both packages are finished installing, open the app.module.ts file under the src/app folder in your Angular app folder structure. Next, we need to import the appropriate Firebase libraries into the Angular app and define which components we need.
Import the AngularFire and Firebase Auth packages:
Import { AngularFireModule } from ‘@angular/fire’
Import { AngularFireAuthModule } from ‘@angular/fire/auth’
Add both import statements to the top of the app.module.ts file in whichever organizational pattern you prefer. Then import the appropriate modules into the Angular app itself. In this case, you’ll need to import the AngularFireModule from ‘@angular/fire’ and the AngularFireAuthModule from @’angular/fire/auth.’
Your Imports object should look similar to this:
Imports: [
…
AngularFireModule.initalizeApp(environment.firebase),
AngulareFireAuthModule,
…
]
Note that the ellipsis in the imports object above should not be added to your code. They only represent whatever entries you may have before or after those import statements.
Final Thoughts
Adding Firebase auth to an Angular app is that easy. Let’s review:
Create a new app in your Firebase dashboard
Copy the Firebase keys to your environments file
Install the Firebase and angular/fire packages via NPM
Import the AngularFireBaseModule and AngularFireBaseAuthModule in your app.modules file
Profit!
Are you looking for a detailed tutorial for Firebase, creating an Angular app, or adding Firebase to an Angular app? Check Shaun Wassell’s Angular and Firebase training course. It’s only a few hours long, and Shaun will show you how to create protected routes, secure Firebase, and much more.
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.